Personal URL Shortener
I recently wanted to make my own URL shortener service, so i could have my own custom short URLs for my social media links or other links i want to share, such as a link to my CV. I figured Cloudflare Workers would be a great way to do this, as it is free and easy to set up. In this post i will be covering how i made my own URL shortener service using Cloudflare Workers, KV and R2.
Pre-requisites
Before setting up your URL shortener service, ensure you have the following things in place as i won’t be covering them here:
- A Cloudflare Account: Sign up or log in to Cloudflare.
- A Domain with Cloudflare: Register a new domain or migrate an existing one to Cloudflare.
What is Cloudflare Workers?
Cloudflare Workers is a serverless application platform running on Cloudflare’s global cloud network of over 200 data centers. It allows you to deploy serverless JavaScript applications across Cloudflare’s global cloud network, closest to your audience.
To create one, head to your Cloudflare dashboard and click on the Workers & Pages tab. You will be greeted with a screen like this:
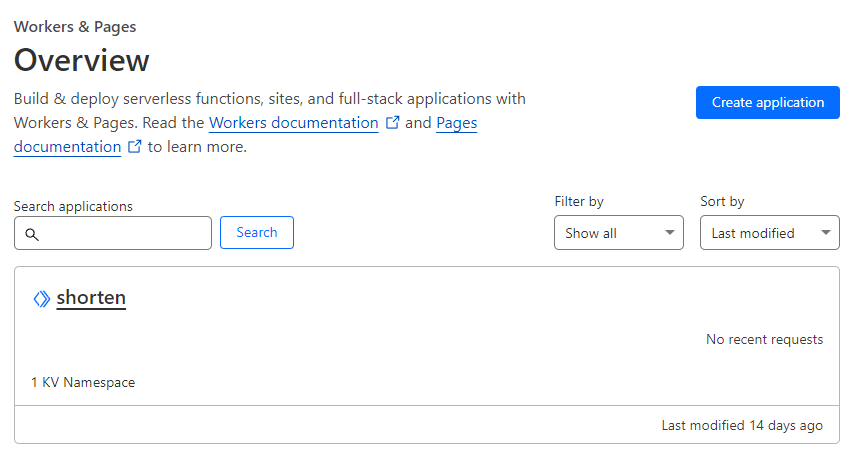
Click on the Create application button and hit the Create a Worker button. You will have to give your worker a name in this step. I named mine shorten
, but now when i think about it, url-shortener
might have been a better name lol.
The name will be deployed to a subdomain of workers.dev
. For example, if you name your worker shorten
, it will be deployed to shorten.[accountID].workers.dev
. You can also add your own domain to the worker which i will cover later.
For now just click on the Deploy button.
After that, you can configure your worker or edit the code.
Storing Data in Workers using KV
Along with the workers platform, Cloudflare also provides a key-value store called Workers KV. It is a globally distributed, eventually consistent, key-value store that spans Cloudflare’s entire network. It is a great way to store data for your workers.
You can create your own KV namespace by clicking on the Workers KV tab in your Cloudflare dashboard and clicking on Create a Namespace and giving it a name.
Hosting files using R2
Cloudflare R2 Storage allows developers to store large amounts of unstructured data without the costly egress bandwidth fees associated with typical cloud storage services.
You can use R2 for multiple scenarios, including:
- Storage for cloud-native applications
- Cloud storage for web content
- Storage for podcast episodes
- Data lakes (analytics and big data)
- Cloud storage output for large batch processes, such as machine learning model artifacts or data sets
Personally i use my shortener for my social media links, but i also have some files hosted on it using R2. For example, i have my CV hosted on it, making it easier to share it with people.
To create one head to your Cloudflare dashboard and click on the R2 tab, and create a Buclet, which is basically a “folder”. Give it a name, set region to Automatic and click on Create Bucket.
You are now able to upload files to your bucket.
But keep in mind that in order to make the files publicly accessible, you need to set the bucket to public. To do that, click on the bucket you just created and click on the Settings tab. Then under Public access find “R2.dev subdomain” and hit Allow Access.
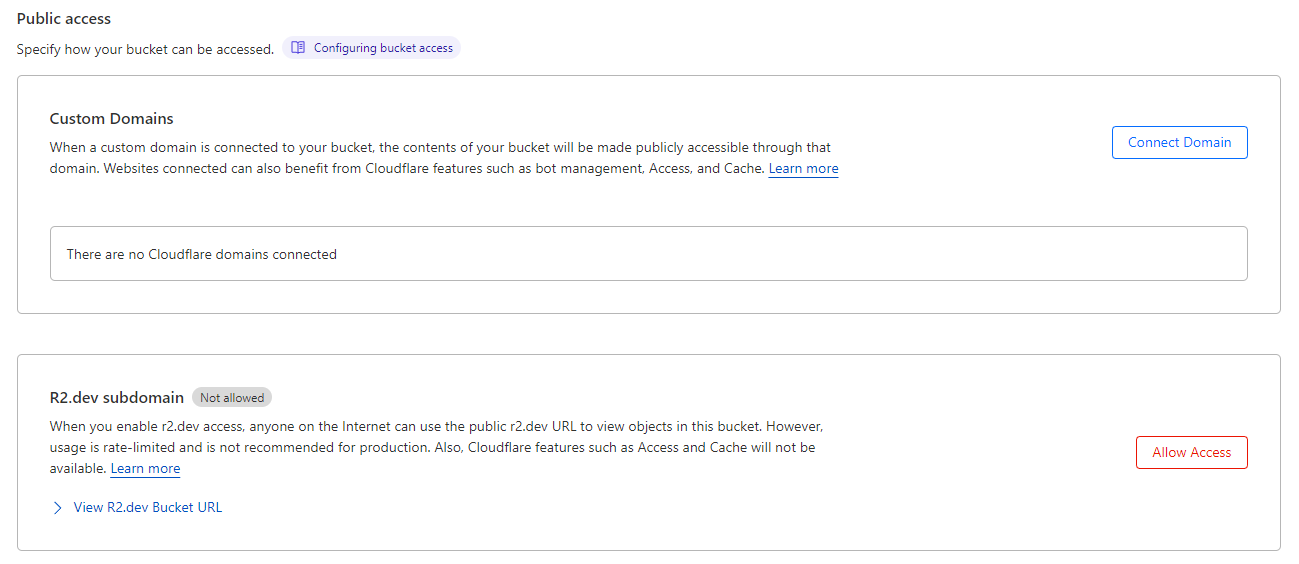
To access files on your bucket such as a file named CV.pdf
you can use the Public R2.dev Bucket URL like this https://[bucket-url].r2.pages.dev/CV.pdf
.
Putting it all together
Now that we have our worker, KV namespace and R2 bucket, we can start writing our code.
I recommend working in a local environment using the Wrangler CLI and then pushing your code to Cloudflare.
Start by installing it using npm:
npm install -g wrangler
Then login to your Cloudflare account:
wrangler login
And initialise a new project from your worker
wrangler init --from-dash shorten
First, we need to import the KV namespace into our worker. To do that, we need to get the KV namespace ID. You can find them in the Workers KV and R2 tabs in your Cloudflare dashboard. It is also possible to create these namespaces using the Wrangler CLI by running:
wrangler kv:namespace create NAMESPACE
This will create a KV namespace with the name NAMESPACE
and return the ID.
Now open the wrangler.toml
file and add the KV namespace ID to the kv_namespaces
array. It should look something like this:
...
kv_namespaces = [
{ binding = "NAMESPACE", id = "INSERT_ID_HERE" }
]
...
To interact with the KV namespace using wrangler you can do something like this:
wrangler kv:key put --binding=NAMESPACE "<KEY>" "<VALUE>"
Or through your worker code like this:
interface Env {
YOUR_KV_NAMESPACE: KVNamespace;
}
let value = await env.YOUR_KV_NAMESPACE.put(key, value);
let value = await env.YOUR_KV_NAMESPACE.get("KEY");
Here you could use the KV namespace to store the URLs and their shortened versions, or even the files you want to host on R2.
Now we can start writing our worker code. I will be using TypeScript for this example, but you can use JavaScript if you want. The shortener will only be able to handle GET requests, so we will need to check for that. We will also need to get the path from the URL, which will be the shortened version of the URL. Then we can use the KV namespace to get the full URL and redirect the user to it.
export default {
async fetch(request: Request, env: any): Promise<Response> {
const url = new URL(request.url);
const path = url.pathname.split('/')[1];
// Route for getting and redirecting to a value by key
if (request.method === "GET" && path) {
const value = await env.shortener_ns.get(path);
if (value === null) {
return new Response("Value not found", { status: 404 });
}
return Response.redirect(value);
}
// Fallback for all other requests
return new Response("Request method not supported", { status: 400 });
},
};
Finally you can deploy your worker using:
wrangler publish
# Or
wrangler deploy
One last thing i want to cover is using custom domains with your worker. To do that, you need to go under your Worker and hit “Triggers”. Here, you can add your custom domain or even subdomain as long as the domain itself is active on Cloudflare.
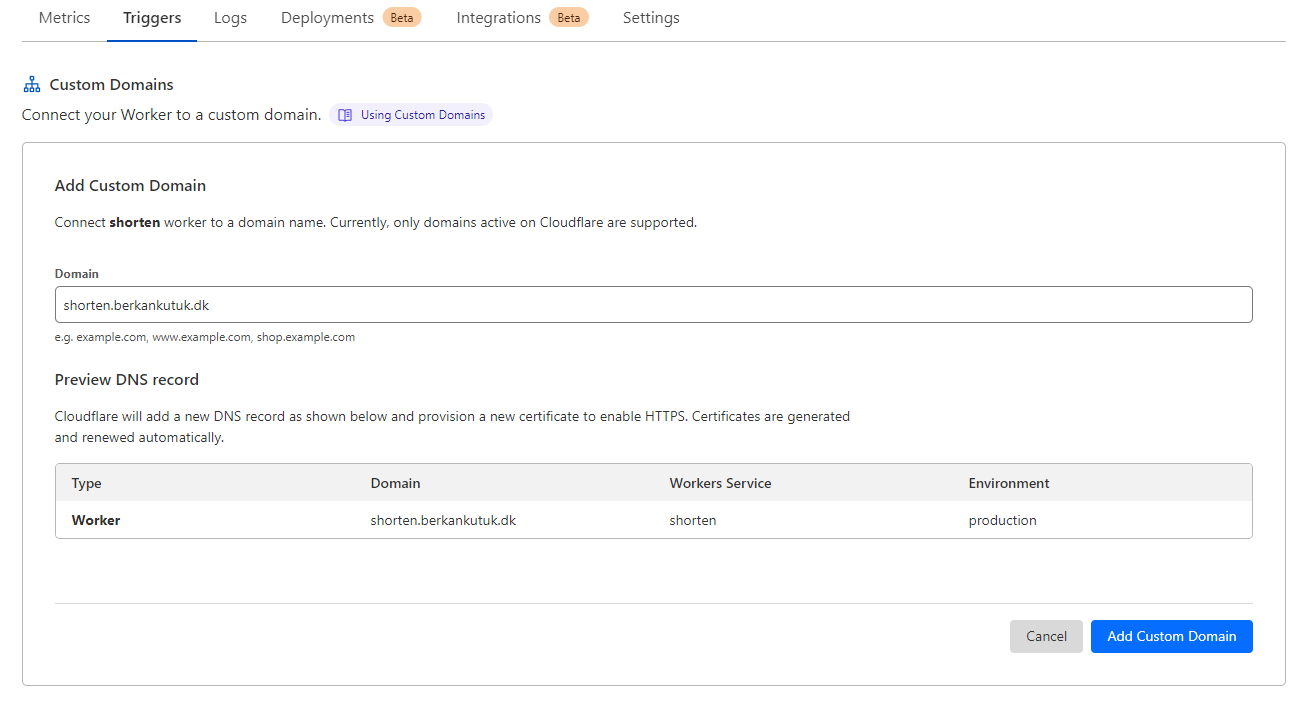
And that’s it! You now have your own URL shortener service. You can use it to shorten your social media links, or even host files on it.
Future Improvements
The next steps from here is fully up to you. You can add a frontend to your worker to make it easier to use, or you can add a simple header-based auth system to prevent others from using your worker. With an auth system in place, you can also add a way to add custom URLs to your KV namespace or even remove existing ones.
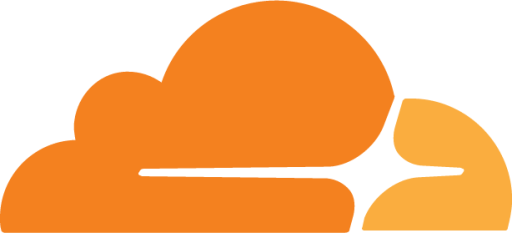